목록C++ (74)
일상 코딩
#include using namespace std; class Cents { private: int m_cents; public: Cents(int cents = 0) { m_cents = cents; } int getCents() const { return m_cents; } int &getCents() { return m_cents; } Cents operator + (const Cents &c2) { return Cents(this->m_cents + c2.getCents()); } }; int main() { Cents cents1(6); Cents cents2(8); // Cents sum; // add(cents1, cents2, sum); cout
#include using namespace std; class Fruit { public: enum FruitType { APPLE, BANANA, CHERRY, }; class InnerClass { }; struct InnerStruct { }; private: FruitType m_type; public: Fruit(FruitType type) : m_type(type) { } FruitType getType() { return m_type; } }; int main() { Fruit apple(Fruit::APPLE); if (apple.getType() == Fruit::APPLE) { cout
1. 익명객체 #include using namespace std; class A { public: int m_value; A(const int &input) : m_value(input) { cout
1. forward declaration 전방선언 #include using namespace std; class B; // forward declaration (전방 선언) class A { private: int m_value = 1; friend void doSomething(A &a, B &b); }; class B { private: int m_value = 2; friend void doSomething(A &a, B &b); }; void doSomething(A &a, B &b) { cout
static은 this-> 포인터 사용 불가. class 내부 함수는 특정 instance에 묶여있지 않다. #include using namespace std; class Something { public: // inner class class _init { public: //inner class 생성자 _init() { s_value = 9876; } }; private: static int s_value; int m_value; static _init s_initializer; public: // 특정 instance 없이도 // s_value에 접근 가능하도록 // 함수 앞에 static을 붙여준다. static int getValue() { //static은 this-> 를 사용하지 못함. re..
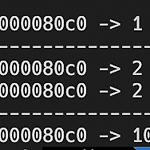
static 변수는 cpp 파일에서만 선언한다. #include using namespace std; class Something { public: static int s_value; }; int Something::s_value = 1; // define in cpp file(static 값일 경우.) int main() { cout
함수 인자로 형태로 넣어주면 같은 메모리를 사용하므로 효율이 극대화 된다. #include using namespace std; class Something { public: int m_value = 0; Something(const Something& st_in) { m_value = st_in.m_value; cout
1. main.cpp 파일 #include "Calc.h" int main() { Calc cal{10}; cal.add(10).sub(1).mult(2).print(); Calc(10).add(10).sub(1).mult(2).print(); return 0; } 2. "Calc.h" header file 선언부만 남겨놓는다. #pragma once #include // 선언부만 남겨 놓는다. class Calc { private: int m_value; public: Calc(int init_value); Calc& add(int value); Calc& sub(int value); Calc& mult(int value); // void div(float value) { m_value /= value..
1. this 포인터 (포인터 주소 확인) #include using namespace std; class Simple { private: int m_id; public: Simple(int id) { // this 는 s1의 포인터 주소. setID(id); cout